Font size
People with a visual impairment often use the accessibility feature to increase the text size. This means that less information than usual is displayed on the screen, so users see less content at once and need to scroll down to read the entire text.
Our accessibility research in the Netherlands shows that more than a fifth of users normally increase the text size on iOS and on Android. When extrapolated to all Dutch people with a mobile phone, we get a figure of more than 3 million people.
Many people make their fonts larger on iOS.
Font-size normal
66%
Font-size larger
22%
Font-size smaller
12%
Our research found that there is a huge diversity of font sizes on Android. The most commonly used setting differs per device type. The default font size therefore seems to differ between the various implementations of the OS. In order to be able to make a statement about the adjustments compared to the standard, we determined per device what the most used setting was and what percentage the font size has changed in relation to this.
Many people make their font-size larger on Android.
Font-size normal
63%
Font-size larger
24%
Font-size smaller
13%
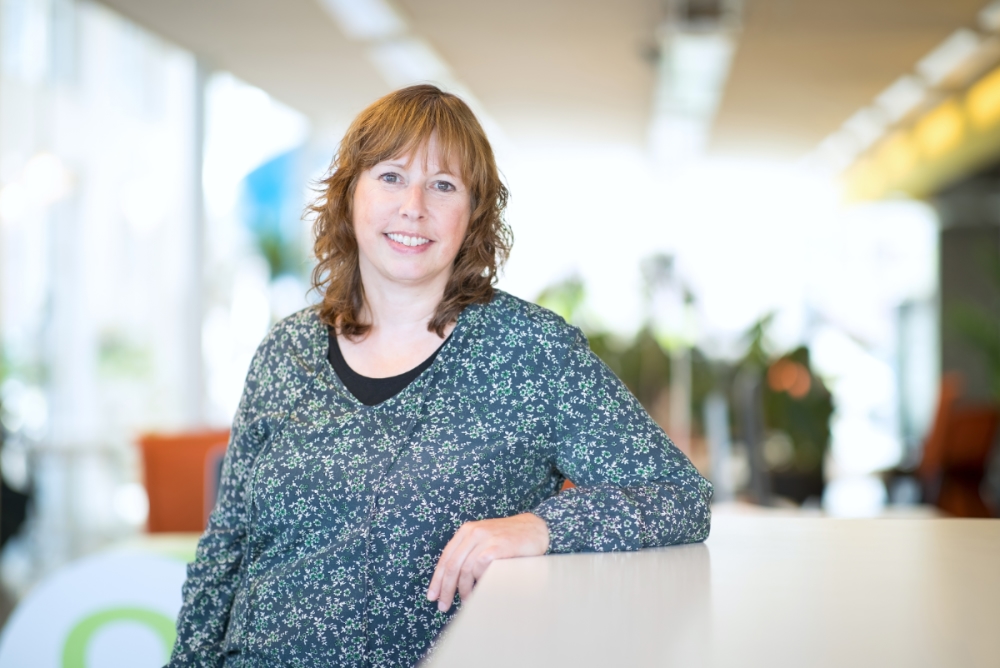
Support in apps
Below are some code samples for the most commonly used platforms and frameworks.
- Android
- Jetpack Compose
- iOS
- SwiftUI
- Flutter
- React Native
- .NET MAUI
- Xamarin
Scale text - Android
On Android, you can use Scale-independent Pixels to scale text. This unit ensures that the user's preferences are taken into account when determining the font size. We recommend to define the textSize
in your styles to make sure it's the same everywhere.
<style name="Widget.TextView">
<item name="android:textSize">18sp</item>
</style>
Scale text - Jetpack Compose
In Jetpack Compose, you can use Scale-independent Pixels to scale text. This unit ensures that the user's preferences are taken into account when determining the font size. We recommend to define the fontSize
property inside the Typography
object in your code to ensure consistency throughout your app.
You can use the @PreviewFontScale
annotation to preview different font scales.
val typography = Typography(
titleLarge = TextStyle(
fontSize = 20.sp,
),
bodyLarge = TextStyle(
fontSize = 16.sp,
),
headlineLarge = TextStyle(
fontSize = 20.sp,
),
)
@FontScalePreviews
@Composable
fun fontScalePreviews() {
Text(text = "This is font scale ${LocalDensity.current.fontScale}")
}
Scale text - iOS
On iOS, you can use Dynamic Type
to scale text. By using this function, the font size is adjusted to the preferences of the user. If you're using your own font, you can use the scaledFont
method from UIFontMetrics
to calculate the font size.
Text elements such as UILabel
, UITextField
and UITextView
have a property called adjustsFontForContentSizeCategory
. If you set it to true
, the element automatically updates its font when the device's content size category changes.
For adjustsFontForContentSizeCategory
to take effect, the element’s font must be one of the following:
A font vended using
preferredFont(forTextStyle:)
orpreferredFont(forTextStyle:compatibleWith:)
with a validUIFont.TextStyle
A font vended using
UIFontMetrics.scaledFont(for:)
or one of its variants
// MARK: - Scaling custom fonts
import UIKit
extension UIFont {
static func font(name: String, size: CGFloat, style: TextStyle) -> UIFont {
guard let font = UIFont(name: name, size: size) else {
fatalError("Font \(name) does not exist")
}
return UIFontMetrics(forTextStyle: style).scaledFont(for: font)
}
static func openSans(weight: UIFont.Weight, size: CGFloat, style: TextStyle) -> UIFont {
if UIAccessibility.isBoldTextEnabled {
return font(name: "OpenSans-Bold", size: size, style: style)
}
switch weight {
case .regular:
return font(name: "OpenSans-Regular", size: size, style: style)
case .semibold:
return font(name: "OpenSans-SemiBold", size: size, style: style)
case .bold:
return font(name: "OpenSans-Bold", size: size, style: style)
default:
fatalError("Font weight \(weight) is not supported")
}
}
}
// MARK: - Enabling content size category adjustments
label.adjustsFontForContentSizeCategory = true
Scale text - iOS
In SwiftUI, scaling text to match the user's preferred content size is straightforward. SwiftUI automatically supports Dynamic Type
, which means your text will adapt to the user's preferred font size set in the device settings.
Text("Appt")
// Scales text automatically
.font(.title)
You can also customize the scaling behavior if you're using custom fonts or want more control. Use the custom(_:size:relativeTo:)
modifier method to ensure the text scales properly.
var body: some View {
Text("Appt")
.scaledFont(name: "Roboto-Regular", size: 24)
}
struct ScaledFont: ViewModifier {
var name: String
var size: CGFloat
var relativeTo: Font.TextStyle
func body(content: Content) -> some View {
content
.font(.custom(name,
size: size,
relativeTo: relativeTo))
}
}
extension View {
func scaledFont(name: String,
size: CGFloat,
relativeTo: Font.TextStyle = .body) -> some View {
self.modifier(ScaledFont(name: name,
size: size,
relativeTo: relativeTo))
}
}
Scale text - Flutter
Flutter automatically scales the text on the screen to the text size set by the user. We recommend using ThemeData
to use the same text sizes and fonts everywhere.
Try to avoid using the textScaleFactor
property because it overrides the text scale factor preferred by the user. The default factor is 1.0
, but can go as high as 4.0
for some users. Restricting the number means that some users might not be able to read the text.
There are valid use cases to restrict the textScaleFactor
to a certain number. You can use MediaQuery
to override the value globally. You can also override it for a single use case by using the property inside a Text
widget.
MediaQuery(
data: MediaQuery.of(context).copyWith(
textScaleFactor: 1.0, // Override scale for all widgets
),
child: ...,
);
Text(
'Appt',
textScaleFactor: 1.0, // Override scale for a single widget
);
Scale text - React Native
React Native automatically scales text depending on the font size preferences of the user settings. In addition, all dimensions in React Native are unitless, and represent density-independent pixels.
Try to avoid using properties such as maxFontSizeMultiplier
, allowFontScaling
, adjustsFontSizeToFit
and numberOfLines
. Using these properties may cause text to be unscalable or become inaccessible.
When inheriting a project you may find previous developers have disabled font-scaling with the following code: Text.defaultProps.allowFontScaling = false;
. This is accessibility anti-pattern and should be rolled back.
The code example below shows how to have a scaling font size.
<Text style={{ fontSize: 16 }}>
Appt
</Text>
Scale text - .NET MAUI
In mAUI, all controls that display text automatically apply font scaling. The scale is based on the font size preference set in the Android or iOS operating system.
By default, .NET MAUI apps use the Open Sans
font on each platform. However, this default can be changed by registering additional fonts in your app.
You can find additional guidance in the .NET MAUI fonts article.
<Label Text="Appt"
FontSize="18"
FontAutoScalingEnabled="True"
FontFamily="Custom" />
Scale text - Xamarin
In Xamarin Forms you make styles for the scalable fonts that you use in your app.
First, accessibility scaling should be enabled for named font sizes. This can be done by pass True
to the the SetEnableAccessibilityScalingForNamedFontSizes
method of the Application
. This can also be done in XAML by using ios:Application.EnableAccessibilityScalingForNamedFontSizes="true"
.
Secondly, you have to register the font and it's properties with the assembly. Afterwards, the fonts can be used in your app and they will automatically scale depending on the users' font size preference.
For more information, see Understand named font sizes, Named font size scaling and Dynamic Styles.
The code examples below shows how to enable font size scaling and how to use dynamic styles.
using Xamarin.Forms;
[assembly: ExportFont("Lobster-Regular.ttf", Alias="Lobster")]
[assembly: ExportFont("Lobster-Bold.ttf", Alias="LobsterBold")]
namespace Project
{
public partial class App : Xamarin.Forms.Application
{
On<Xamarin.Forms.PlatformConfiguration.iOS>().SetEnableAccessibilityScalingForNamedFontSizes(true);
}
}
<Application
xmlns:ios="clr-namespace:Xamarin.Forms.PlatformConfiguration.iOSSpecific;assembly=Xamarin.Forms.Core"
ios:Application.EnableAccessibilityScalingForNamedFontSizes="true">
</Application>
<Style TargetType="Entry">
<Setter Property="FontFamily" Value="Lobster" />
</Style>
<Style
x:Key="LabelRegular"
ApplyToDerivedTypes="True"
BaseResourceKey="BodyStyle"
TargetType="Label">
<Setter Property="TextColor" Value="Black" />
<Setter Property="FontFamily" Value="Lobster" />
<Setter Property="FontSize" Value="{DynamicResource Body}" />
<!-- For Android you have to set FontSize property -->
</Style>
<Style
x:Key="LabelBold"
ApplyToDerivedTypes="True"
BaseResourceKey="LabelRegular"
TargetType="Label">
<Setter Property="FontFamily" Value="LobsterBold" />
<Setter Property="FontAttributes">
</Style>