An Accessible App. Where to start?
Making your app accessible to everyone: where do you start? Below are the main steps that will help you make your app usable for the widest possible group of people, including people with disabilities.
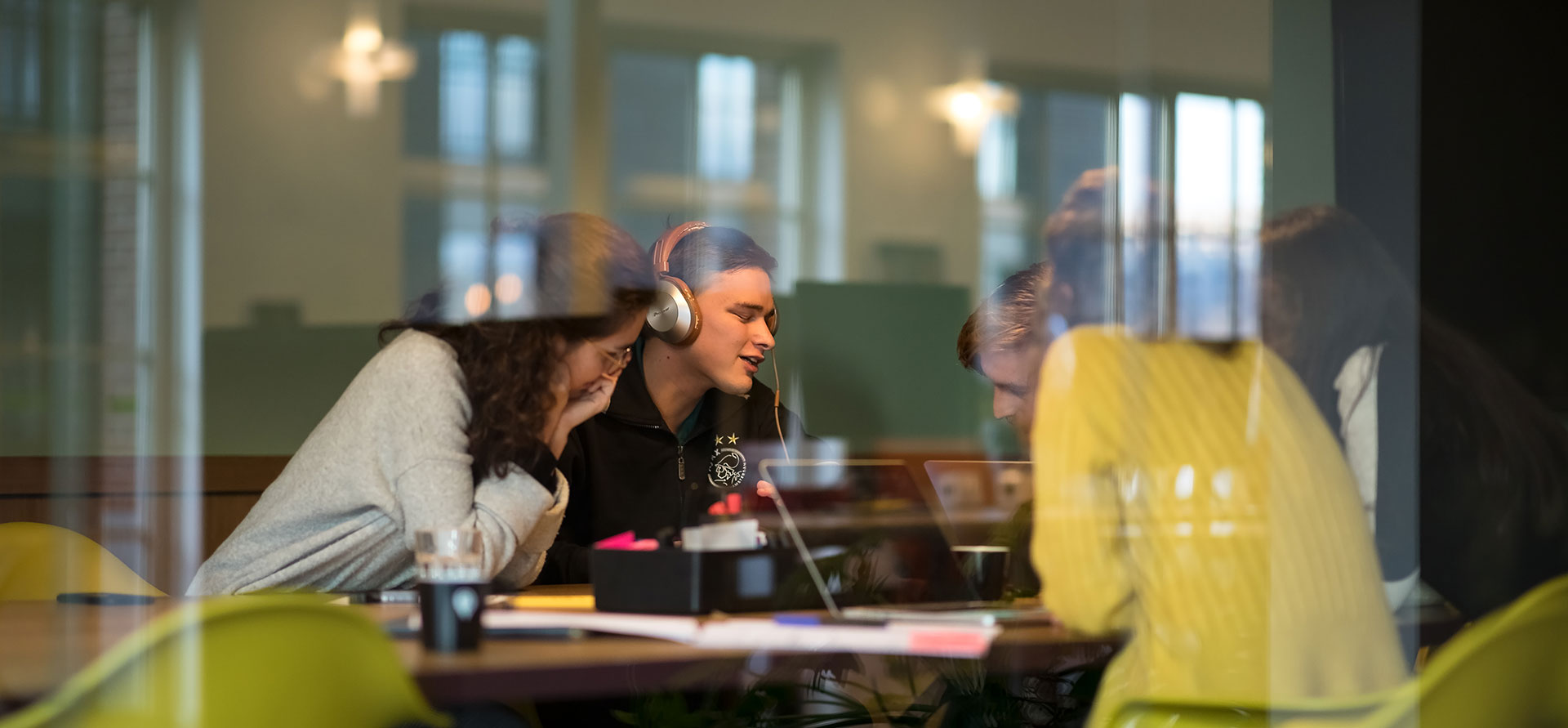
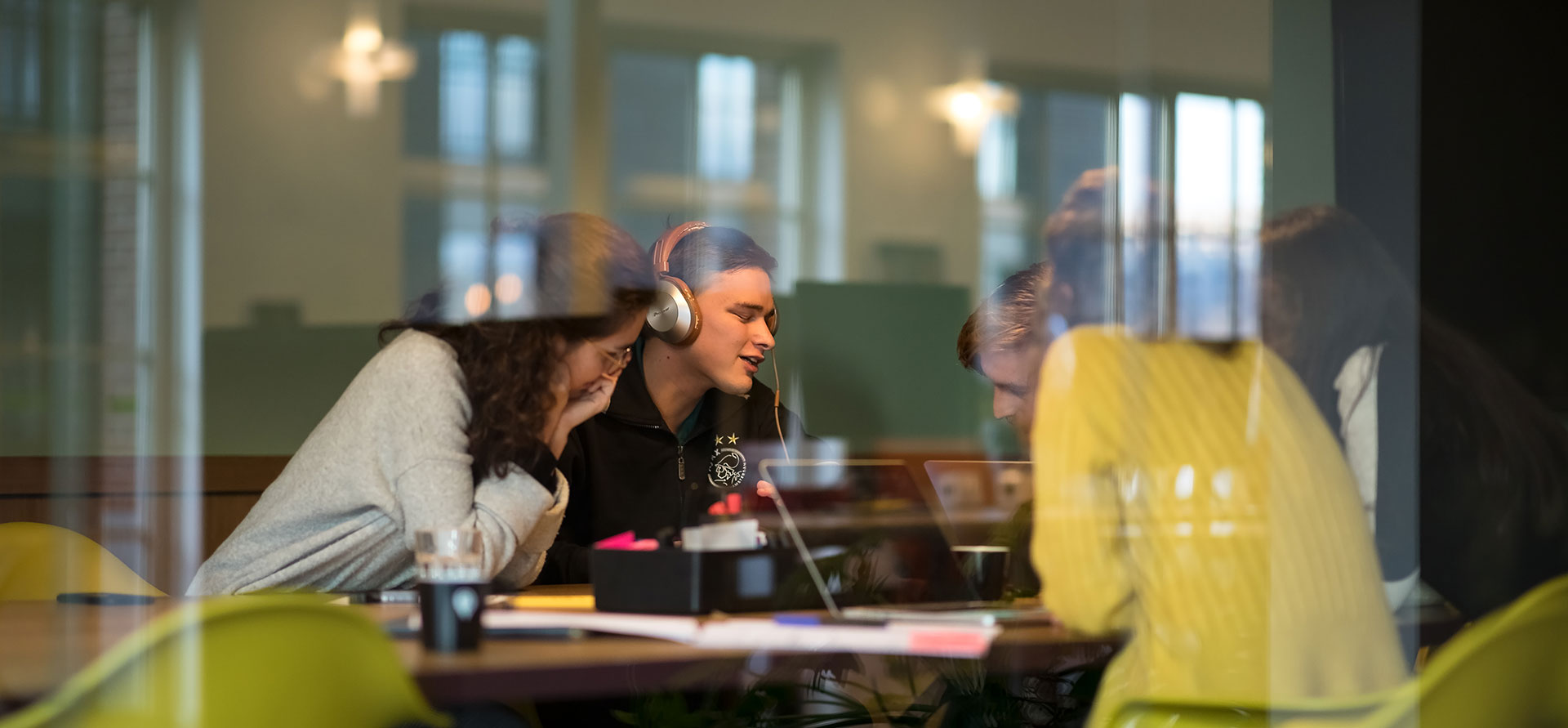
It can seem very complicated and even intimidating when you delve into the vast amount of information on digital accessibility. How do you incorporate all those guidelines, insights and code examples into an app? To get you started, we have listed the six most important steps for you.
Use a screen reader!
Offer textual alternatives.
Make it clear what the purpose is.
Make sure text can be resized.
Provide sufficient contrast.
Show focus and offer a logical order.
Below is a detailed explanation of each step. Finally, we explain how you can test whether it works as intended.
Note that this list is not exhaustive and will not make your app fully accessible. But is meant to make the biggest possible difference through a few simple steps.
1. Use a screen reader!
To properly test whether your app is accessible, it pays to learn how to use a screen reader.
A screen reader is mainly used by visually impaired people. However, by optimising your app for this, you ensure that a much larger group of people can use your app. Users of voice, keyboard and switch controls will also benefit, and you automatically take into account a lot of success criteria from the WCAG.
This makes the screen reader the ideal tool to test for accessibility. But it can take some getting used to. It all works a little differently from what you are used to. With the ScreenReader app, you learn all the gestures and possibilities as you go.
2. Offer textual alternatives
Do all buttons have a clear label? Do icons, images, graphics, among others, have a textual alternative?
Make sure alternative text is available for all content without text. These include images, icons and graphs. Describe what can be seen. People who are blind have this description read aloud through their screen reader. Alternative text can also be useful for anyone who is unsure about the meaning of the content.
You do this by setting an accessibility label. You can see how to do this for your app in the example below.
3. Make clear what the purpose is
Is it clear what action can be performed?
Set a name. The name is used for identification. Setting a name allows tools such as voice control to perform targeted actions.
Set accessibility name
Set a role. With the role "button", it is clear that an action takes place on activation. With the role "link", it is clear that you are directed to another location. Setting a role makes it clear to tool users what they can do.
Set accessibility role
Set a value. For a checkbox, the value is "selected" or "not selected". For a volume control, the value can be "50%". By setting a value, this can also be passed textually to tools.
Set accessibility value
4. Make sure text can be resized
Is it possible to display text in a larger font size? Does text remain readable with a larger font size?
Ensure that the text in your app supports resizing. Users specify their preferred font size in the system settings. Text in your app should resize according to the preferred font size. This is especially important for visually impaired users because otherwise they might not be able to read the text. Text should not be abbreviated with dots.
Support text scaling
Ensure that all content on the screen remains readable even with the largest font. Content should be readable without having to scroll in two directions. Because the text is displayed larger, it can push other elements off the screen. Ensure content can still be reached, for example, by scrolling vertically.
Prevent text truncation
5. Provide sufficient contrast
Does text have sufficient contrast relative to the background? Do elements on the screen have sufficient contrast against each other and in relation to the background?
Ensure that the content on the screen has a contrast of at least 3:1 with the surrounding color. Think of graphic elements such as icons, buttons and input fields.
Ensure that the contrast ratio between the text color and background color is at least 4.5:1. For bold and large text, a ratio of 3:1 is sufficient.
By maintaining these ratios, visually impaired and color blind users can usually read the text well. In addition, this makes an app easier for everyone to use, for example outside in the sun.
6. Show focus and offer a logical order
Is it clear where the focus is? Is the order in which the focus moves logical?
Ensure that elements focused by assistive technologies are clearly indicated. Focus is often shown by placing a box around the element. Make sure that the placement is correct and that the color is clearly visible. For apps it is not possible to adjust the color of the frame. However, it is possible to give elements a different background color when they have focus.
Add accessibility focus indicator
Ensure assistive technologies use a logical focus order when navigating. The order of navigating a screen is usually from left to right, from top to bottom. Make sure assistive technologies use an equivalent focus order.
Adjust order for keyboard
Adjust order for assistive technologies
Move accessibility focus
Testing
You can test most of the steps with the Accessibility Scanner app and Xcode's Accessibility Inspector. You can also detect contrast issues automatically with these. another option is to take a screenshot of an app to determine the color codes and calculate the contrast. This can be done with WebAIM's Contrast Checker, for example.
In addition, check that it works properly by using the screen reader.
To test whether text scaling is properly supported, set a large font size. You can do this on a phone under the accessibility settings.
Questions?
By following these steps, you will make your app accessible to a very large group of people. Anything missing? Is something not quite clear? Let us know in the Slack channel of Appt.